Getting Started With React
Are you looking at getting started with React but don’t know where to start? Don’t worry – you’re in the right place!
Let’s dive right in.
Disclaimer: before you read this and want to jump right in, here are a couple of things you should probably know (or at least be familiar with) first:
- Javascript. React is a Javascript Library so you’re gonna need to understand at least the basics. I’d recommend getting started at MDN Web Docs.
- NodeJS. For the way I’m going to set up the example React App in this tutorial, you’ll need to have NodeJs installed on your computer and have at least a basic understanding of how NPM works. But don’t let this over face use – we’ll just be using NodeJS and NPM to quickly install everything we need (and more) to get a quick install of React working (more on this later).
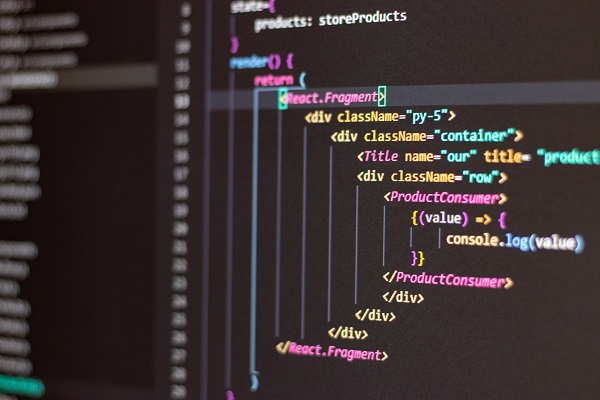
What is React?
React is a really popular web technology right now – and rightly so.
React is an open-source Javascript library that’s used for building quick User Interfaces (UI’s).
React was created by Facebook software engineer Jordan Walke as was first deployed on Facebook news feeds in 2011. Since then it’s been adopted on many big websites such as Instagram, Paypal, Netflix, and many more.
Why Use React?
One of the reasons why React has become so popular is that there are many advantages to using it:
- Getting started with React is quick & easy.
- It promotes a component-based design – that means code is written to be re-used and therefore building user interfaces is faster.
- Allows developers to logically see the flow of data.
- It uses a Virtual Dom which allows for better performance when it comes to user interaction and data updates.
- A special mashup of HTML and Javascript called JSX makes it easier to write code. It also makes the code easier to read and using JSX prevents XSS attacks, so it also makes the code safer. See more information about this in the React Documentation.
- ReactJs.org has great documentation and tutorials for you to follow to better understand how everything works.
Some of those advantages may not make sense to you right now and that’s okay.
Just wait until you start using it; then everything will click into place and you’ll fall in love with it just like I have.
Now that we know what React is and why it’s a good idea to use it, let’s actually take a look at getting started with React.
Getting Started with React: How to set up React
There are a few of ways to set up React:
- Import into your HTML file like any other Javascript library (note: you’ll need a couple of other libraries to get it working though – more on this later).
- Use something called Create-React-App to install a quick start React environment.
- Use Webpack (and a few other tools, libraries, etc) to create your own React environment. Don’t worry about this one for now. It’s a bit advanced for our purposes in this getting started with React article, we’ll get into this somewhere down the line.
Adding React to a Webpage like a Library
The fastest way to add React to a Webpage is to use the HTML Script tag to load into the page like any other Javascript file.
Here’s a CodePen with a working example:
See the Pen Loading React Directly into the Web Browsers by Kris Barton (@krisbarton) on CodePen.
There’s lots to unpack here.
Firstly, we need to load more packages to get it working. These packages are:
- ReactDom.
- Babel.
What is ReactDOM?
You don’t have to worry too much about this and how it works, just that you need it.
But if you want a brief explanation, the ReactJs.org documentation does a pretty good job of explaining it:
“The react-dom
package provides DOM-specific methods that can be used at the top level of your app and as an escape hatch to get outside of the React model if you need to.”
Essentially, it’s an efficient way of handling DOM elements of a webpage.
In the example above, we use ReactDOM’s render method to add a component (more on that later) to our div element (with the id of “root”).
TL;DR – Don’t worry about how ReactDOM works, just know that we’re using it and it’s pretty useful.
What is Babel?
In a nutshell, Babel is a tool that React uses to compile its code to standard Javascript so that web browsers can understand it.
Drawbacks of Setting Up React this Way
Using React this way is absolutely fine functionality-wise.
But for usability and readability? Not so much. For really small apps you might not come across any issues. For bigger apps, enhancing and updating that code is not going to be pleasant – it’s going to get out of control pretty quickly and will basically make your life harder.
So what’s the “right” way to set up React? There is no real “right” way but using a build environment makes it a whole lot easier and cleaner.
So how do you use React with a build environment? The quickest way is to use Create-React-App. Here’s how.
What is Create-React-App?
Create-React-App is basically an “out of the box” React build environment. You install this and BAM! you have a pre-made React app that contains dummy content.
This tool was created by the developers at Facebook as way for developers new to React to quickly learn how to use it.
It’s also great for prototyping – a quick start React app, full of features and functionality that’s ready to go.
Essentially, if you’re just getting started with React you should use Create-React-App.
How to set-up Create-React-App?
Create-React-App is installed on the command line and uses NodeJs and NPM (node package manager – it’s built into NodeJs).
So the first step in setting up Create-React-App is making sure you have NodeJS installed or, if you already have NodeJS installed, making sure you have the right version installed. You can check what version you’re currently using, type node -v into a command line.
You will need to have version 8.10 or higher installed in order to use this create-react-app installer.
If you don’t already have NodeJs installed on your computer, you can download NodeJs here and install it like you would any other program.
So, what do you do to actually install create-react-app?
You install create-react-app in a command line. You can use any, but my preference is the terminal in VSCode. It’s my Code Editor of choice, so it just makes sense.
In your command line, you install create-react-app by typing the following:
npx create-react-app my-app
This goes and gets the create-react-app code and installs in a folder called my-app (if that folder doesn’t exist, one will be automatically created). And yes, you can change the folder name to whatever you’d like.
Then type this in the terminal:
cd my-app
All this does is get your terminal into the my-app folder. This is so that we can run the next bit of code, which we’ve installed in this my-app folder.
Obviously, if you’ve gone ahead and called your folder something else, change the command above to reflect that. So cd “whatever your folder is”.
Finally, we want to get the react app running.
npm start
This triggers a function in the package.json file that initializes the application. Don’t get bogged down on this bit if you don’t fully grasp what’s going on here – the more you use stuff like this, the more it will fall into place.
All you need to know for now is: it starts the React environment for you to view in a web browser.
So, if you go to http://localhost:3000/ in your web browser of choice, your app will be running and you’ll see a React welcome page.
Now that our React app is up and running, let’s take a look at some React coding concepts.
Getting Started with React: Coding Concepts
I know what you want to do.
Now the app is set up, you want to go ahead, pull it apart, and do some coding. I know, because that’s exactly what I’d want to do.
But before you do, you should check out some of core coding concepts that come with React – it’ll make your life a whole lot easier, trust me.
There are 5 main concepts:
- JSX.
- Elements.
- Components.
- Props.
- State.
Let’s take a look at each of them in a bit more detail.
JSX
One of the first things you’ll come across when building apps in React is JSX.
What is JSX?
JSX stands for Javascript XML.
It’s a Javascript syntax expression used mostly in React. It basically allows us to easily write HTML within Javascript.
For a basic example of this:
const hello = <h1>Hello, world!</h1>;
As you can see, we’re declaring a variable as we normally would in Javascript, but the syntax after that is not common: it’s neither a string nor HTML…
…it’s JSX.
JSX isn’t essential for developing in React, but it does make life a whole lot easier.
For example, without JSX the above code snippet would need to be:
const hello = React.createElement('h1', {}, 'Hello, world!');
I think you’ll agree, just by looking at it JSX is clearly easier to write.
Expressions in JSX
JSX also allows us to write expressions and embed them in the code.
For example, what if we wanted to add introduction text to that Hello, World! greeting?
const greeting = "My name is Kris Barton.";
const hello = <h1>Hello, World! {greeting}</h1>
ReactDOM.render(
hello,
document.getElementById('root')
);
Let’s see this in action in CodePen:
See the Pen JSX Expressions by Kris Barton (@krisbarton) on CodePen.
With Expressions, you can embed pretty much anything from function output or equations like 2 + 2…it’s really cool.
JSX Can Used in Logic
Because JSX is compiled to Javascript, logic may be used to render elements.
What does this mean? We may use if and for statements to determine output.
For example:
function doGreeting(user) {
if(!user.name){
return <div>Hi there.</div>
}
return <div>Hi, my name is {user.name}</div>;
}
How to Specify an Attribute in JSX
One more important thing you need to know about writing JSX code are attributes.
Much like HTML attributes may be written and assigned to a JSX element.
For example, consider this image element:
const user = {
name: "Kris Barton",
avatar: "img.jpg"
}
const avatar = <img src={user.avatar} alt="This is the user's avatar" tabIndex="0" className="avatar__img" />
There’s a bit to unpack here.
Firstly, you will note tabIndex and className.
In regular HTML, this would be tabindex and class respectively. Because JSX is closer to Javascript than HTML, ReactDOM uses the camelCase naming convention.
But don’t worry: it will get compiled to the attribute name you’ll be expecting by the time it get’s to a web browser.
Next you will probably note the attributes take two different types of value: a string and a value in curly braces.
Just remember:
- Attributes can take both strings and expressions.
- These attribute curly braces work in the same way as our greeting example above – as JSX Expressions. So, in the example above the <Img /> element’s src attribute will compile to <img src=”img.jpg” alt=”This is the user’s avatar” tabindex=”0″ class=”avatar__img” />
For more information and examples of JSX, check out the React docs and for a little more advanced JSX stuff, check out the Advanced JSX React docs.
Now let’s move onto the next React coding concept: Elements.
Elements
The React documentation goes a great job of describing what elements mean in React:
“An element describes what you want to see on the screen“
So, from my first JSX example above, the following is an element:
const hello = <h1>Hello, world!</h1>;
The same as the below chunk of code is an element:
const list =
<ul>
<li> Item One </li>
<li> Item Two </li>
<li> Item Three </li>
</ul>
And as you’ve seen from the CodePen examples above, we render an element by using ReactDOM’s render method.
const greeting = "Hi, my name's Kris Barton.";
const hello = <h1>Hello, World! {greeting}</h1>;
ReactDOM.render(hello, document.getElementById('root'));
As you can see, we:
- Call the ReactDOM’s render method.
- Passes in our element as the first argument.
- Then we tell it where to render the element. In this case, in an HTML element that has the id of root.
Now let’s take a look at slightly bigger concept that’s important for getting started with React: components.
Components
The concept of components isn’t unique to React, but it’s a big part of React’s DNA.
A component-based architecture allows developers to create reusable chunks of code that can easily be reused throughout a website, an app or even on the same web page.
It allows use to create code in isolation that does not need to interact with out components or aspects of the web page they’re on at all.
Again, the ReactJS.org documentation perfectly describes components in React:
“Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen.”
How to Define a Component in React
In React, component’s can be defined a couple of different ways.
The first way is pretty much exactly like defining a Javascript function (don’t worry about props just yet, we’ll cover that next):
function Hello(props) {
return <h1>Hey there, {props.name}.<h1>;
}
The second way to define a component is using Javascript ES6:
class Hello extends React.Component {
render() {
return <h1>Hey there, {this.props.name}.</h1>;
}
}
How to Render a Component in React
We render a component in React pretty much how we’d rendered an element in the example earlier in the article.
function Hello(props) {
return <h1>Hey there, {props.name}</h1>;
}
const greeting = <Hello name="Kris Barton" />;
ReactDOM.render(
greeting,
document.getElementById('root')
);
Let’s break down the example above:
- We declare Hello as a component just like we would a regular Javascript function.
- We use the props object to grab the name attribute and use JSX expressions to add it to the welcome message. More on props later.
- Then, we use it like we would an HTML element. We declare the name attribute and give that attribute a value.
- Then we render the component in our root div using the ReactDOM.render() method.
Components within Components
As mentioned earlier in this section, components allow us to break down our User Interface into small chunks.
So, in React we have the ability to use components within components and of course use the same component multiple times.
So using the example above, we abstract our Hello element and add it multiple times (with different names) to another function. Then, we’ll use ReactDOM to render everything.
That looks a little like this:
function Hello(props) {
return <h1>Hey there, {props.name}</h1>;
}
function App() {
return (
<div>
<Hello name="Joe Montana" />
<Hello name="Jim McMahon" />
<Hello name="Terry Bradshaw" />
</div>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
Let’s take a look at a working version of that code:
See the Pen Rendering Components in React by Kris Barton (@krisbarton) on CodePen.
I think that covers components pretty well, let’s move onto Props.
Props
We’ve already already used examples of Props earlier in the article and by now you’ll probably have a good idea what they are.
Here’s a quick Props overview:
- Props stands for properties.
- Props are arguments that are passed into React Components, which are then used in those React Components.
- Props are immutable. Meaning they’re read-only – we can display and use them, but not alter them.
- Props are just regular Javascript objects.
Let’s take one of the components we created and passed props into in the last Components example.
<Hello name="Joe Montana" />
Here, we declare an iteration of the Hello component and pass name into the component as props.
Inside the component, we have access to the value of name by accessing props.name. Like this:
function Hello(props) {
return <h1>Hey there, {props.name}</h1>;
}
The naming is like for like: so we declare the attribute as name and access in the props as props.name. If we decided that we wanted to add an age attribute, we’d declare it like this:
<Hello name="Joe Montana" age="64" />
And access it using props.age. So let’s apply this to the component:
function Hello(props) {
return <h1>Hey there, {props.name}. I'm {props.age} years old.</h1>;
}
Now let’s take a look at that in our CodePen.
See the Pen Adding the Age Props by Kris Barton (@krisbarton) on CodePen.
Essentially…
You can think of props as an object in which of the component attributes are stored.
Now it’s time to look at another important concept that you absolutely need to know when getting started with React.
State
What is state in React? Here’s a quick overview:
- State is a Javascript object that stores all of a component’s dynamic data and determines behaviour.
- When the state object changes, the component re-renders.
- State can only be used in a Class component out of the box. For a functional component, React Hooks may be used instead.
- The state handles the component that it’s declared and used in.
Sounds a bit like a prop, right?
What’s the difference between Prop and State?
I think the explanation from the React Documentation is the best:
“
props
(short for “properties”) andstate
are both plain JavaScript objects. While both hold information that influences the output of render, they are different in one important way:props
get passed to the component (similar to function parameters) whereasstate
is managed within the component (similar to variables declared within a function).“
I think a good demonstration of state within a component is a Timer function.
Consider the below React component.
class Timer extends React.Component {
constructor(props) {
super(props);
this.state = {date: new Date() };
}
render() {
return <h1>Current time: {this.state.date.toLocaleTimeString()}.</h1>;
}
}
ReactDOM.render(
<Timer />,
document.getElementById('root')
);
A couple of things to note:
- When using a constructor, props have to be passed in using super(props) – don’t worry about this just yet.
- We declare the state in the constructor using this.state = {date: new Date()}.
- We display the name using a JSX expression pretty much the same way we did props with this.state.date.
- We render the component in the exact same way as before – passing it into ReactDOM.render() and pushing it our div with an id of root.
The example above captures the state on page load and renders the output – in this date the current time.
But state can be updated within a component. Most commonly, this is through user interaction – a button click, a form input or something like that.
And to do this, we use the this.setState() option.
So:
- We use this.state on initialization in the construction function. i.e. this.state.date = new date().
- We use this.setState() to update the state. i.e. this.setState( date: new date()).
So let’s update our example above so we change update the time to update in real time.
class Timer extends React.Component {
constructor(props) {
super(props);
this.state = {date: new Date() };
}
componentDidMount() {
this.timerId = setInterval(
() => this.updateTime(),
1000
);
}
componentWillUnmount() {
clearInterval(this.timerId);
}
updateTime() {
this.setState( { date: new Date() } )
}
render() {
return <h1>Current time: {this.state.date.toLocaleTimeString()}.</h1>;
}
}
ReactDOM.render(
<Timer />,
document.getElementById('root')
);
Let’s walk through the code a little.
You’ll notice:
- We’ve added a function called updateTime() in which we update the state of the component. i.e. we set it to the current time.
- We’ve added a React Lifecycle method called componentDidMount(). This is a part of the React library and it’s basically a function that fires when the component is mounted i.e. when the page is first loaded.
- In componentDidMount() we’ve set up a standard Javascript setInterval function that fires our updateTime function every second.
- We also used the componentWillUnmount React Lifecycle method that clears the interval.
Don’t worry about the React Lifecycle stuff just yet – I’ll cover that in another article later. All you need to know is that the state updates as intended.
Here’s a working CodePen showing this functionality in action:
See the Pen Using State in React by Kris Barton (@krisbarton) on CodePen.
Are You Set For Getting Started With React?
Hopefully this article has given you a good idea for getting started with React, but remember: the learning doesn’t stop here.
There’s a lot more to learn with React, but this article is a great start.
You might be asking yourself: what’s next? What’s next for learning React?
Next Steps for getting started with React are:
- Read through the React Documentation from back to front.
- Once you’ve installed create-react-app as mentioned earlier in this article, pull it apart and try to see how it all hangs together.
- Come back and check out more React articles here.
Further Reading
Below is a list of resources we’ve used in our Getting Started with React article:
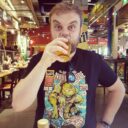