Javascript Remove From Array
You want to use Javascript to remove a value from an array, but you can’t quite remember how. You think the answer should be something obvious and simple, yet you can’t quite put your finger on what it actually is.
Perhaps array.remove() is a thing? You think, hopefully. That would be REALLY useful..
Guess what. It’s not a thing and that, quite understandably, frustrates you.
You think, Well, what the hell should I do then?
Let’s take a look at your options.

Javascript Remove First Item from Array with the Shift Method
Before we get into some of the more complex methods of removing items from an array, it’s probably a good idea to start with the basics – removing the first item of an array.
This can be achieved quite easily with the Javascript Shift method. Let’s take a look at an example:
Let items = [‘item1’, ‘item2’, ‘item3’];
items.shift(); // will get you [‘item2’, ‘item3’]
And to add an item to the start of the array, you use the unshift Javascript method. Let’s take a look at that in action:
Let items = [‘item2’, ‘item3’];
items.unshift(‘item1’); // will get you [‘item1’, ‘item2’, ‘item3’]
Pretty straightforward stuff, right? Now let’s take a look at removing the last item from an array.
Javascript Remove Last Item from Array with the Pop Method
As you might have already guessed, this Javascript method is just as straightforward as the shift method.
It’s called pop and it works in the exact same way as shift. Let’s take a look:
Let items = [‘item1’, ‘item2’, ‘item3’];
items.pop(); // will get you [‘item1’, ‘item2’]
To add an item back to the end of the array, you use the push method, like this:
Let items = [‘item1’, ‘item2’];
items.push(‘item3’); // will get you [‘item1’, ‘item2’, ‘item3’]
But removing an item from anywhere within the array? That’s where things get a little bit more complex.
Javascript Remove from Array with Splice Method
We’ll start with the most common method for removing an item from an array: splice.
What is the Javascript splice method? The MDN Web Docs definition is:
The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place.
You basically use the splice to determine three things:
- Which part of the array you want to change – it’s index.
- How many items in the array you want to remove.
- The new item you want to insert into the array.
So the syntax for the splice method is like this:
array.splice(index, howmany, replacement items);
So let’s take a look at a standard splice method in action. The scenario is, we have an array of items and we want to replace the second item in the array with a different item.
Let items = [‘item1’, ‘item2’, ‘item3’];
items.splice(1,1,’item4’); // Will get you [‘item1’, ‘item4’, ‘item3’]
Here, I’m telling the splice method to index 1 and replace 1 item with the ‘item4’ value.
But wait. If you’re starting at index 1, why is it the second item in the array that’s being replaced rather than the first? You might be thinking.
Good question. It’s because the array index actually starts at 0, not 1. So in our array, it would go 0 = ‘item1’, 1 = ‘item2’, 2 = ‘item3’.
Let’s test this by changing the 1 to a 0 in our splice method.
Let items = [‘item1’, ‘item2’, ‘item3’];
items.splice(0,1,’item4’); // Will get you [‘item4’, ‘item2’, ‘item3’]
Complex Array Splicing
We’ve used the Javascript splice method to replace an item in an array, but what if you just wanted to remove an item? What then?
Well then you just don’t stipulate the replacement item.
Let items = [‘item1’, ‘item2’, ‘item3’];
items.splice(1,1); // Will get you [‘item1’, ‘item3’]
Pretty straightforward so far, right? So why can this be complex?
What if you wanted to remove an item from an array, but didn’t know the index of the item within the array? It’s a likely scenario, especially when dealing with dynamic content.
So how can we accomplish this? We’ll need to do three things:
- We’re going to need to loop through the array.
- We’re going to need to check the value we want to remove against all the values in the array.
- If we find the value in the array, we need to remove it from the array.
Let’s take a look how we can do this:
let moreItems = [1, 2, 3, 4, 5];
let removeItem = 3;
for (i = 0; i < moreItems.length; i++) {
if(moreItems[i] === removeItem) {
moreItems.splice(i,1);
}
}
// will get us [1,2,4,5]
Okay, let’s run through what I’ve done here.
First of all, I’ve defined our array and then I’ve defined the item we want to find in our array and remove.
Then I’ve run through the array using the Javascript for loop. I’ve created a variable, called it i and set its initial value to 0. Then I’ve told the loop to run through the array until the end of the array, which I’ve dynamically determined using the .length Javascript method. Then I’ve set the loop to increment my i variable by 1 for every loop until it reaches the length of the array.
Inside the loop, we’re using our i variable as an index for our variable and then comparing each array value to the value we’re searching for.
If we find a match, we use that i variable as our splice index and remove it from our array.
Then the loop continues, because you never know when there might be more of those values we want to remove.
Let me demonstrate by adding more items to the array.
let moreItems = [1, 2, 3, 4, 5, 2, 6, 7, 3, 8, 9, 6];
let removeItem = 3;
for (i = 0; i < moreItems.length; i++) {
if(moreItems[i] === removeItem) {
moreItems.splice(i,1);
}
}
// will get us: [1,2,4,5,2,6,7,8,9,6]
But our fun with removing items from a array doesn’t end there. There is, in fact, a slightly better way handle item removal with the Javascript filter method.
Javascript Remove from Array with Filter Method
The Javascript filter method accomplishes what our splice method inside a FOR loop did, just with slightly less lines of code and without actually having to use a for loop. The filter method also allows for a little more added cool functionality in case we wanted to be a little clever.
First, let’s take a look at how we can use the filter method to remove items from an array.
let moreItems = [1, 2, 3, 4, 5, 2, 6, 7, 3, 8, 9, 6];
let removeItem = 3;
moreItems = moreItems.filter(item => item !== removeItem);
// will get us: [1,2,4,5,2,6,7,8,9,6]
With the Javascript filter method, that’s literally all there is to it.
Let’s take a look what’s going on here:
- We’re re-assigning our array to contain the filtered items.
- Then the filter method will search through each item in our array and we declare a variable for each item (in the example above, we call it item, but it could be called anything you like) and compares it against the value we want to remove.
- If the item is not equal to the item we want to remove, we keep it.
One other thing to note about the example about is the use of the Javascript Arrow function (=>). If you’re unfamiliar with it, the Arrow Function isn’t a part of the Javascript Filter method – it’s actually the new fangled way of writing functions in ES2015 Javascript. Therefore, this piece of code will not work in Internet Explorer at all (but it does work in Edge). For more information on what browsers do currently support this, check out CanIUse.
So, the above ES2015 written code is basically this in old Javascript:
moreItems = moreItems.filter(function(item){
return item !== removeItem;
});
Makes sense, right?
There are also a few extra cool things you can do with the Javascript method which might appeal to your array item removal needs – you can set conditions.
For example, say we only wanted items in the array that was bigger than 3. We could do this:
var biggerThan = function(value) {
return value > 3;
}
moreItems = moreItems.filter(biggerThan);
// will get us [4,5,6,7,8,9,6]
Here we create a function that accepts a value and returns the value if it’s bigger than 3. We pass this function into our filter method and the filter method knows to access the biggerThan function with each item in our array and filter out the unwanted values accordingly.
Pretty cool, right?
Conclusion
So what’s the best way of removing items from an array?
Personally, I prefer using the filter function over the splice function because it’s less code and I think it’s a nicer way to go about things. BUT, if I was ever in a situation where I just wanted to remove the first or last item from my array, I’d use the shift and pop Javascript methods accordingly.
I guess my point is, the best way to use Javascript to remove items from an array depends on what exactly you need to do and your situation.
Further Reading:
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter#Polyfill
- https://www.w3schools.com/jsref/jsref_splice.asp
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice
- https://alligator.io/js/push-pop-shift-unshift-array-methods/
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter
- https://www.w3schools.com/jsref/jsref_filter.asp
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions
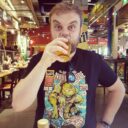